Create JSON data in javascript
Creating JSON data in JavaScript is straightforward. JavaScript has a built-in object called JSON
that includes methods for converting JavaScript objects to JSON strings and vice versa.
Step-by-Step guide to create JSON data in javascript
- Create a JavaScript object: This object will hold the data you want to convert to JSON.
- Use
JSON.stringify()
: This method converts the JavaScript object to a JSON string. - Output or use the JSON data: You can log it to the console or use it in your application.
Code Example
Here’s a complete example of how to do this in JavaScript:
Step 1: Create the JavaScript Object
Define an object with your data:
const data = {
name: "John Doe",
email: "john.doe@example.com",
age: 30,
address: {
street: "123 Main St",
city: "Anytown",
state: "CA",
zip: "12345"
},
phone_numbers: {
home: "555-555-5555",
mobile: "555-555-1234"
}
};
Step 2: Convert the Object to JSON
Use JSON.stringify()
to convert the JavaScript object to a JSON string:
const jsonData = JSON.stringify(data);
Step 3: Output the JSON Data
You can log the JSON string to the console or use it in your application:
console.log(jsonData);
Full Example Code
Putting it all together, the complete JavaScript code looks like this:
// Step 1: Create the JavaScript object
const data = {
name: "John Doe",
email: "john.doe@example.com",
age: 30,
address: {
street: "123 Main St",
city: "Anytown",
state: "CA",
zip: "12345"
},
phone_numbers: {
home: "555-555-5555",
mobile: "555-555-1234"
}
};
// Step 2: Convert the object to JSON
const jsonData = JSON.stringify(data);
// Step 3: Output the JSON data
console.log(jsonData);
Explanation
const data
: Defines a JavaScript object with the data you want to convert.JSON.stringify(data)
: Converts the JavaScript object into a JSON string.console.log(jsonData)
: Outputs the JSON string to the console.
This JSON string can be used for various purposes, such as sending data to a server via an API, storing it in a file, or passing it between different parts of a web application.
You can view check or confirm JSON data in JSON viewer
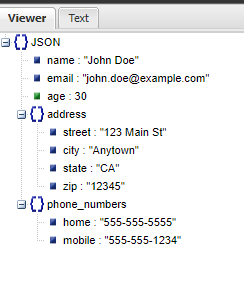